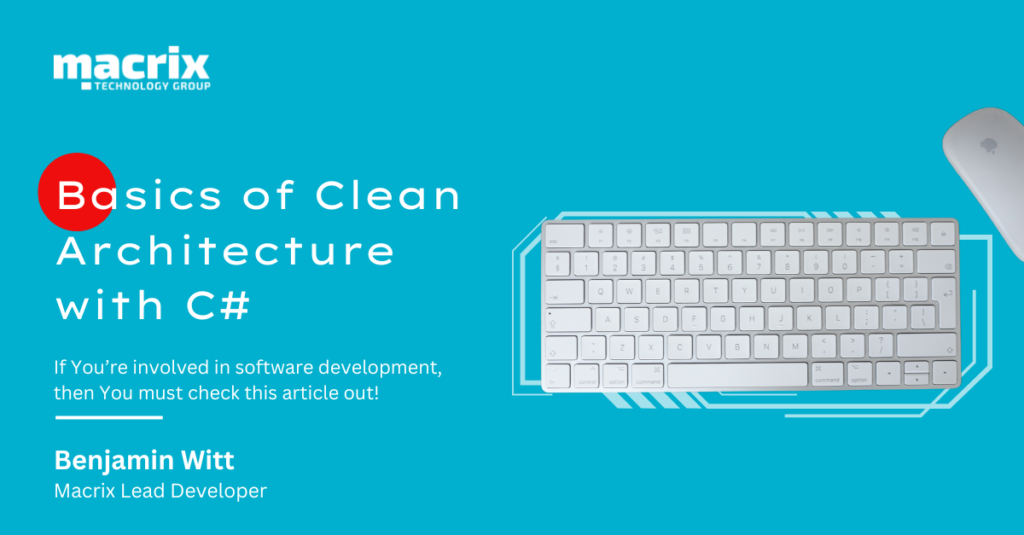
Introduction to Clean Architecture
What is Clean Architecture?
Clean Architecture, proposed by Robert C. Martin or “Uncle Bob,” is an architectural pattern for software projects aimed at keeping the code clean, modular, and independent of external influences. It isolates the business logic of the application from external details such as user interfaces, databases, and frameworks.
Why is Clean Architecture important?
Clean Architecture offers a variety of advantages:
High Maintainability:
The clear separation of business logic and technical details facilitates understanding of the code and enhances its maintainability.
Extensibility:
Thanks to the well-structured architecture, new features can be added or existing ones modified easily without jeopardizing the integrity of the overall application.
Testability:
The clean separation of components simplifies the writing of automated tests, thereby improving the quality of the software.
Independence from external influences:
By using abstractions and interfaces, the application remains flexible and is not tied to specific technologies or frameworks.
The benefits of Clean Architecture include:
Clear Separation of Responsibilities: The clear structure defines the responsibilities of the various components, leading to better-organized code.
Minimization of Dependencies: Clean Architecture minimizes dependencies between different parts of the application, enhancing flexibility and maintainability.
Facilitation of Testing: The clean separation of components allows tests to focus on individual parts of the application, improving testability and software quality.
Support for Scalability and Extensibility: The modular structure of Clean Architecture makes it easier to scale the application and add new features without redesigning the entire architecture.
The Principles of Clean Architecture
Separation of Concerns:
This principle demands that various aspects of a software application, such as user interface, business logic, and data persistence, be separated from each other. This leads to better-structured and more maintainable code.
Dependency Rule:
The dependency rule states that dependencies within an application should always point towards stable layers. For example, the business logic layer should not have direct dependencies on the user interface or databases.
Stable Dependencies Principle:
This principle dictates that dependent components should be more stable than the components they depend on. This means that high-level modules should not depend on low-level modules.
Stable Abstractions Principle:
Here, the abstraction should be more stable than the concrete implementation. Abstractions should be independent of concrete implementations.
These principles form the backbone of Clean Architecture and contribute to creating a robust and flexible software architecture. Now, let’s take a look at the components of Clean Architecture.
The Components of Clean Architecture
Clean Architecture comprises various components designed to divide the application into clearly defined layers. Here are the main components:
Dependency Inversion Principle:
This principle states that dependencies should depend on abstract interfaces, not concrete implementations. This decouples the layers and increases the flexibility and testability of the application.
Entities:
Entities represent the core objects of the application, representing the state and behavior of the business logic. They are independent of the database or other external systems.
Use Cases:
Use Cases contain the application logic and define how user interactions are handled. They are independent of the user interface and other external systems.
Interface Adapters:
These adapters connect the application to external systems such as databases, APIs, or user interfaces. They convert data and calls into formats that can be processed by the core application.
Frameworks & Drivers:
This layer contains external frameworks and drivers used by the application, such as web frameworks, database drivers, or user interface libraries.
By clearly separating these components, changes can be made in one area of the application without affecting other areas. This makes the application flexible and easier to maintain.
Implementation of Clean Architecture in C#
Implementing the Project Structure:
- Creating the Core Layer:
- Define Entities and Use Cases.
- Create a folder named “Core.”
- Within this folder, classes for Entities such as “User.cs” or “Product.cs” are created.
- Create interfaces or abstract classes for Use Cases like “IUserService.cs”.
Creating the Application Layer:
- Implement Use Cases.
- Create a folder named “Application.”
- Implement Use Cases as classes that inherit from the interfaces defined in the Core layer.
- For example: “UserService.cs”.
Creating the Infrastructure Layer:
- Implement Interface Adapters.
- Create a folder named “Infrastructure.”
- Implement concrete classes for the interfaces defined in the Core layer.
- For example: “UserRepository.cs” for “IUserRepository”.
Implementing Entities:
Entities represent the core objects of the application and include the state and behavior of the business logic. Here’s an example of a “User” Entity:
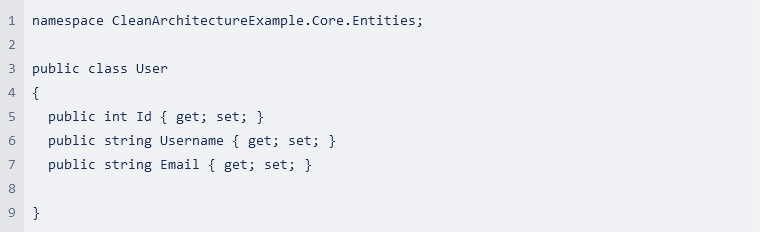
With this structure, we lay the foundation for a clean and modular architecture that is easily maintainable and extensible.
Implementing Use Cases:
Use Cases contain the application logic and define how user interactions are processed. Here’s an example of a “UserRegistration” Use Case:
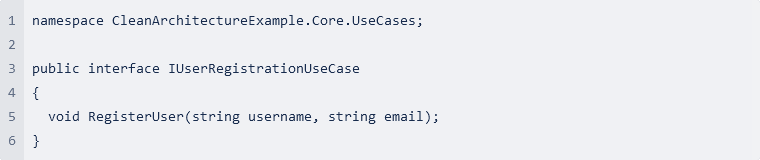
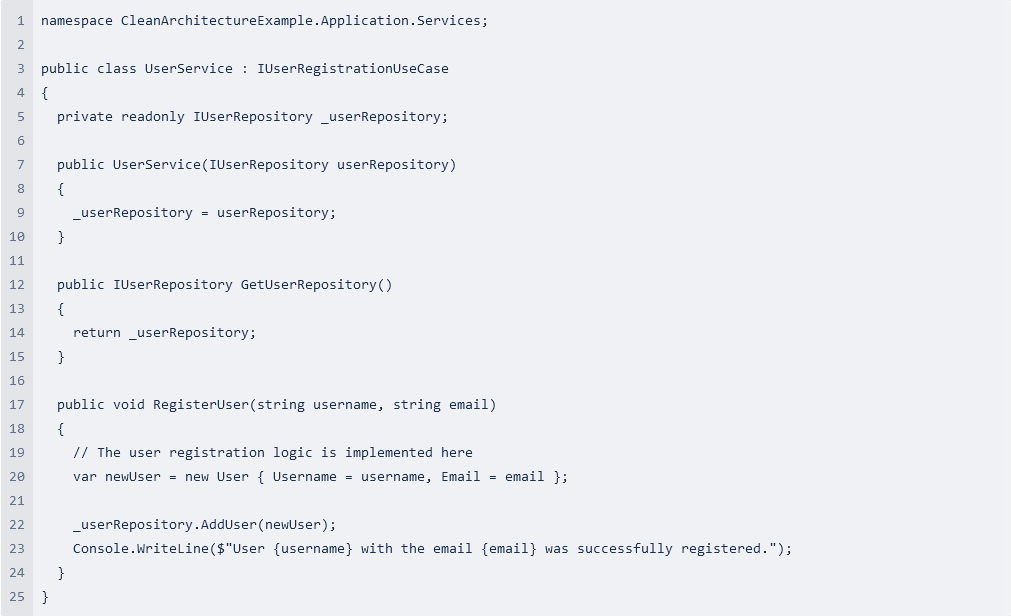
Implementing Interface Adapters:
Interface Adapters connect the application to external systems such as databases or APIs. Here’s an example of a UserRepository implementing the database access interface:
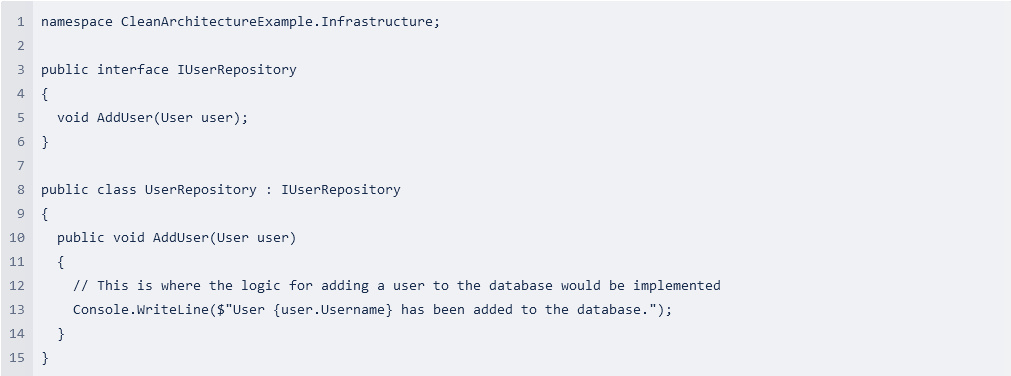
Now, we will proceed to manage dependencies between components using Dependency Injection.
Setting up Dependency Injection:
In this step, we will set up Dependency Injection (DI) in our C# project to manage dependencies between different components.
First, we need to configure a DI container in our project. We can use the built-in DI container library provided by .NET for this purpose. Here’s an example of how we can do this:
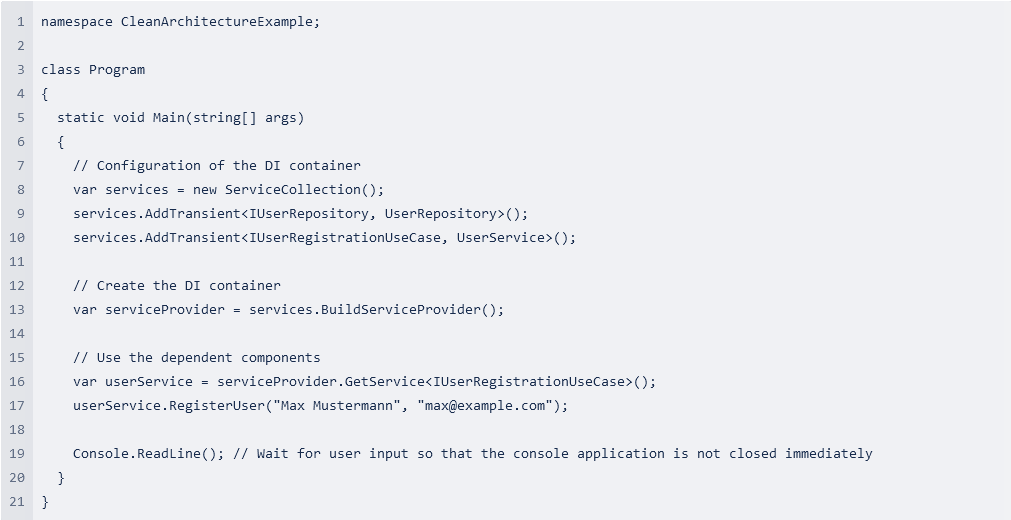
In this example, we use the `ServiceCollection` class to register dependencies. With `AddTransient`, we specify that a new instance of the dependent class should be created each time it’s requested.
Once we have configured our DI container, we can use the dependent components in our classes. The DI container takes care of resolving and providing the dependent instances.
For example, the `UserService` can access the `IUserRepository` like this:
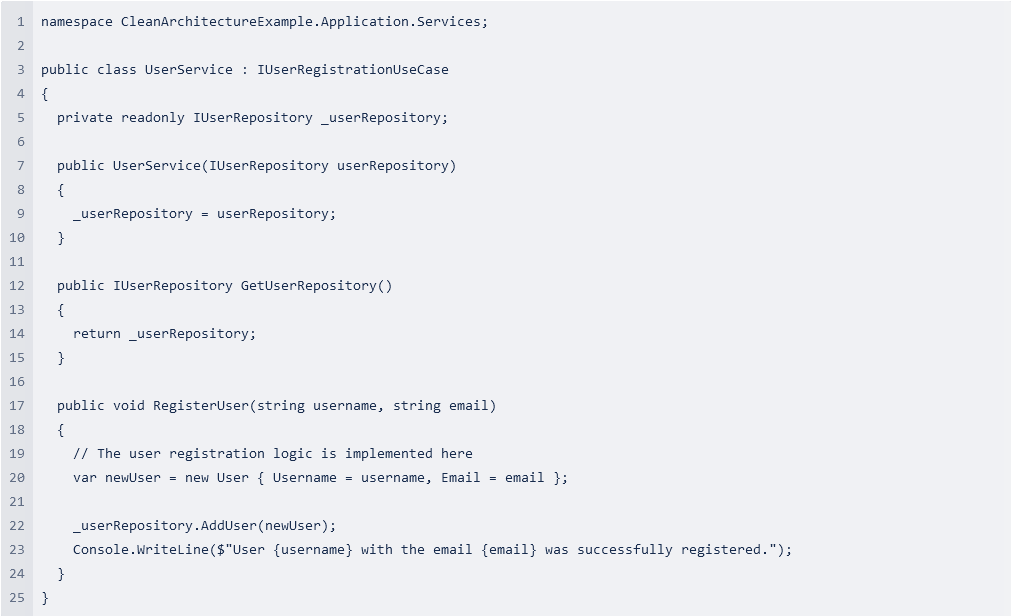
By using Dependency Injection, the components are loosely coupled, which improves the maintainability, extensibility, and testability of our application.
Testing in Clean Architecture
Testing is an integral part of Clean Architecture, ensuring that our application functions correctly and that changes do not introduce unwanted side effects. In Clean Architecture, we use various types of tests:
Unit Tests:
These test individual components of our application in isolation. Mock objects can be used to simulate external dependencies.
Integration Tests:
These test the collaboration of multiple components of our application to ensure they interact properly.
End-to-End Tests:
These test the entire application from an external perspective, such as by executing user interactions through the user interface.
Test-Driven Development (TDD) Approach:
In the test-driven development approach, we first write tests that define the expected behavior of the application, and then implement the code that passes these tests. This approach promotes thorough test coverage and often leads to better code quality and structure.
Example Tests for Various Components:
Here is an example of a unit test for the `UserService`:
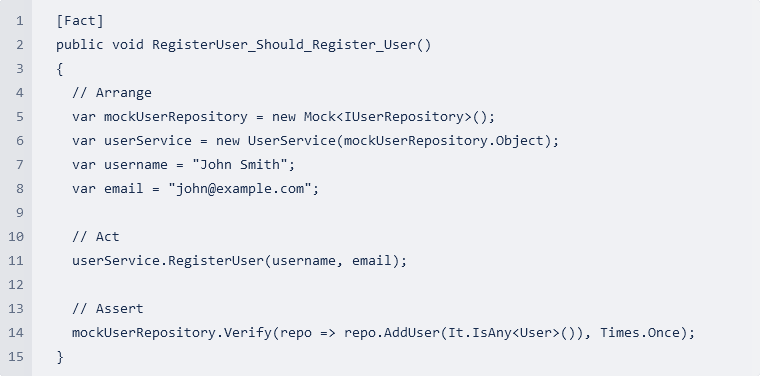
In this test, we use a mock object for `IUserRepository` to simulate database access. We verify that the `AddUser` method of the repository was called once after registering a user.
Conclusion:
In this article, we’ve provided a comprehensive overview of the fundamentals of Clean Architecture with C#, covering everything from basic principles to practical implementation and testing. Clean Architecture offers a multitude of benefits for software project development, including improved maintainability, extensibility, and testability. By clearly separating responsibilities and minimizing dependencies, the code becomes more structured and easier to maintain. The use of Dependency Injection allows for flexible configuration of the application and facilitates testing by isolating components. Overall, Clean Architecture provides a robust approach to developing high-quality software that has proven itself in a variety of application domains.
Benjamin Witt
Macrix Lead Developer
Ps. For more of Ben’s publications along with exercise files and demos, go here.
Useful links:
1. A quick introduction to clean architecture
3. Understand clean architecture in 7 minutes
4. Clean architecture in ASP>NET Core Web API